What Is The JavaScript ParseInt Function?
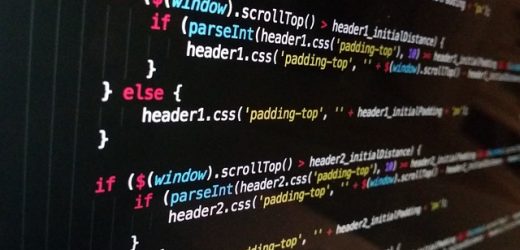
JavaScript is a powerful programming language that thrives on the web. It comes with a number of functions that make programming websites and web application simple. A useful function Javascript provides is the parseInt() function.
The JavaScript parseInt() function parses a string and returns an integer value. This function is useful for times where you want to convert data a user has inputted as a string to an integer. The applications for parseInt() are numerous, and it will become a valuable tool in your programming toolset.
We will walk you through how the parseInt() function works, practical applications of the function, technical information, and some common mistakes programmers make when using the parseInt() function.
How To Use The JavaScript ParseInt() Function |
In order to explain what the parseInt() function is, and how it works, we will break up the information into the following sections:
- Overview
- Constructor
- Use
- Examples
- Return values
Overview |
The parseInt() function parses a string and either returns a number, or NaN(Not a number) if the string read in does not contain a valid number. In a perfect world, you would only deal with solid numbers that can easily be converted into integers, like 10, or 500.
However, you may find times where there are numbers that you want to convert to a string that are represented as hexadecimal numbers. Hexadecimals aren’t the only numbers you have to concern yourself with, there are also octals and other number bases that you may need to convert.
Thankfully, the parseInt() function is versatile and accepts parameters that specifically help programmers convert strings to numbers based on radix. This is important work done by the constructor of the parseInt() function.
Constructor |
The parseInt() function takes the following parameters:
- String
- Radix
The String you want to parse is a required parameter in this function.
The radix parameter is an optional parameter that specifies what numeral system needs to be used for the conversion process. If you were to input a radix of 16, the parseInt() function would interpret the number you are parsing from the string as a hexadecimal.
The radix parameter can be any value in between 2 and 36. Because the radix parameter can be omitted, it is important to know what default value is used. When there is no radix parameter defined there are a few assumptions JavaScript makes to interpret the string.
If the string begins with “0x” then the radix interpretation will be 16. Otherwise, any other value provided to the parseInt() function will be interpreted with the radix of 10. It is important to know this going into using the parseInt() function in case you run into miscalculations of integers you want to parse.
When you start using the parseInt() function, it is important that you get in the habit of defining your radix. This is especially true if you are working with large data sets that have the possibility to deviate. Creating a function that shifts the radix based on expected number value in a string is well worth your time.
Use
Because JavaScript parseInt() is a function, you usually want to set it to a variable. This way you can store the integer value returned by the function. Common usage of the parseInt() function is as follows: var a = parseInt(“10”);
Examples
The parseInt() function can convert many different string values to integers. Regular numbers, octals, and hexadecimals are among some of the most common conversions used with the parseInt() function. The following examples are common uses of parseInt():
- parseInt(“10”) returns 10
- parseInt(“010”) returns 10
- parseInt(“17”, 8) returns 15
- parseInt(“C”, 16) returns 12
- parseInt(“-0XC”, 16) returns -12
Notice that if you define the radix value as 16, you can use characters that are not numerals without throwing a NaN error.
Return Values |
When the parseInt() function is used correctly it returns an integer. However, there will be times when the parseInt() function cannot correctly parse a string, in those cases, it will return a NaN error.
It is important that you pay attention to the return value provided to you by parseInt() and create an exception that handles the NaN error.
If the first character in the string cannot be converted to a number, then the Javascript parseInt() function will throw a NaN error. Likewise, if you supply an empty string, the parseInt() function will return nothing.
Applications Of Javascript ParseInt Function |
The parseInt() function is a powerful tool that has a number of practical applications. The following applications of the parseInt() function demonstrate its practicality:
- Sanitize user inputs
- Convert octal and hexadecimal values
- Format Microsoft JSON Date
Sanitize User Inputs |
The primary application of parseInt() is to sanitize user inputs. There may be moments where you have users input text into a text box. Any time the user inputs text into a text box, that value will be referred to as a string.
In order to pull the integer value you desire from the string, you must use the JavaScript parseInt() function. Getting comfortable with this function will better prepare you to deal with a common interaction paradigm on the web.
Convert Octal And Hexadecimal Values |
Javascript’s parseInt() function isn’t just useful for sanitizing user inputs, it is also useful as a conversion tool. There may be times where you are given hexadecimal or octal values as strings. No one wants to have to look up a key every time they see one of these values.
Unless you have octal or hexadecimal values memorized, or you understand the conversion, the parseInt() function can do much of the heavy lifting in converting those values into human readable integers. You might be creating a website component that relies on strictly integer values. The best way to interpret those values would be to use the parseInt() function.
Format Microsoft JSON Date |
Another key practical application of parseInt() is for use as a date converter. There will be times where you have to format Date information to make more human-readable text. In a popular example on StackOverflow with over 674,333 views a user explains how to use the parseInt() function to sanitize date data.
Notice that in most examples parseInt() is used to make information more readable. Practical applications of the parseInt() function generally make the use of other functions easier. Knowledge of regular expressions will help you better use of this function.
Technical Information |
Image Source: Unsplash.com
The JavaScript parseInt() function is usable on all modern web browsers. Basic support is available for all web browser platforms, and each browser supports parsing leading zero strings.
This function also has 3 specifications that can easily be read for more technical information on the parseInt() function. The following specifications exist for parseInt():
- ECMAScript 1st Edition
- ECMAScript 5.1
- ECMAScript 2015
- ECMAScript Latest Draft
Another technical case that is important to understand is how different versions of ECMAScript numeric strings with leading zeroes that do not have defined radixes. ECMAScript3 discourages the use of parsing octals with no radix defined. In ECMAScript 5, parsing an octal without defining the radix as 8 will definitely not work. It is important that you always define your radix when using the parseInt() function on octal and hexadecimal values.
Common Mistakes |
One of the most common mistakes programmers make when using JavaScript parseInt() , is not setting their radix. A user may parse a string that has a leading zero and receive output that is different from what they are expecting. This Stack Overflow statistic demonstrates that this is a common error with over 14,880 views over 7 years.
To solve this mistake, it is imperative that you understand how parseInt() parses strings when no radix is supplied. Always supply a radix, it is better to be explicit when using the parseInt() function.
The ParseInt() Function Is A Necessary JavaScript Tool |
Javascript’s parseInt() function can be used in a number of instances to make JavaScript development easier. Common uses of this function involve parsing strings to convert numbers into an Integer format that can easily be manipulated.
It is important that you remember to define the radix. If you don’t define your radix you could run into issues using the parseInt() function that can slow down your development time. As always, consult StackOverflow or Quora if you run into bugs that you cannot fix on your own!
Featured Image Source: Pixabay.com
What do you think?