What Is An Insertion Sort Algorithm – Its Basic Definition
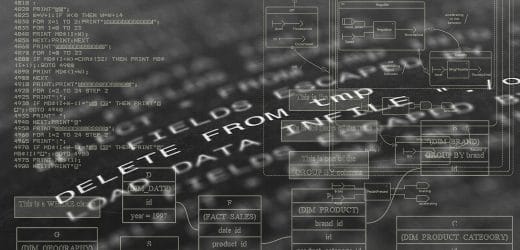
If you need to get a good understanding of what an insertion sort algorithm is, the best way to start is with a basic definition of what an algorithm is.
An algorithm in its purest sense is just a formula or method for solving a problem. Even a simple task may include an algorithm by utilizing a standard process for arriving at a solution. This could include a variety of types of problems, and their associated resolutions:
Modern computer applications are where insertion sort algorithms enter the picture. In computer science and mathematics, an algorithm is a defined specification that eases the burden of solving even complex problems.
By formalizing a process or function as a proven algorithm, programmers and scientists can reuse code and formulas to solve business and mathematical problems more efficiently.
Computer algorithms are essentially program logic that receives input values and produces consistent, reliable results as output. Algorithms can be applied for automated and consistent reasoning, performing calculations, and yes – sorting.
Types of Sorting
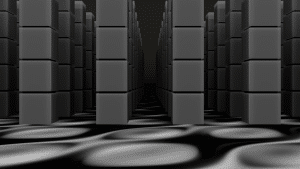
There are multiple methodologies and algorithms for conducting computer sorting:
Even within those variations in processing, and the applicable uses for each, there are additional classifications such as recursive insertion sort, binary insertion sort, recursive merge sort, and so on.
Insertion Sort Explained
So just what is an insertion sort algorithm?
Insertion sort algorithms work much in the same way as you would in sorting a deck of cards. Assume someone gives you stack of playing cards, already in order (or even a single card). Then they give you another card, asking you to place it in the proper sequence in the deck. You will scan through the deck you have, then insert the new card in its place.
Next, you’re given another card, with the same request – put in the deck – in sequence. With many iterations of cards passed to you, the process is repeated. This is essentially the process in working with an insertion sort algorithm.
For each iteration, processing is required to shift the array to insert the new entry, which can be an important factor in utilizing an insertion sort when large arrays or data sets are anticipated. In effect, the insertion sort algorithm proceeds in this manner:
This provides a reasonably straight-forward process, yet also reveals how the algorithm can result in considerable processing, when the input set is composed of extremely large arrays.
Variations of an Insertion Sort
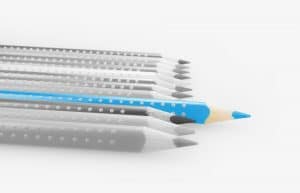
Within the realm of insertion sort processing, there are additional variations:
Binary insertion sort - binary insertion sort can be used to reduce the actual number of comparisons over a normal insertion sort. By utilizing a binary search function to insert an element in the proper position of the output set, less processing is required. Normal insertion sort will require multiple iterations for comparison, depending on the size of the input array. In a worst case of large arrays, the binary insertion sort can have significant performance advantages.
Recursive insertion sort–insertion sort algorithms can also be written recursively, although this could have a negative impact on performance. Recursion can simplify coding of the algorithm, but can increase processing requirements.
Insertion sort methodology is more commonly implemented in a non-recursive manner.
Insertion Sort Algorithm Characteristics/Caveats
One factor of sorting algorithms is the attribute of being termed stable or unstable. This refers to the occurrence of equal values in array elements, and whether the sequence of those elements will be retained in the same order as originally encountered in the output set. Insertion sort algorithms are stable by their very nature.
Divide and conquer – algorithms that implement a divide and conquer methodology process data elements utilizing a somewhat more complex approach:
As divide and conquer algorithms require multiple steps, they are recursive in their processing methodology. Where large sets of data are involved, this type of algorithm can provide an advantage in run times (time complexity).
Insertion sort is not a divide and conquer algorithm, processing elements in a single pass.
Why Would You Use (or Not Use) an Insertion Sort Algorithm?
With the many variations of sort algorithms, why would you decide you use the insertion sort algorithm for any particular problem?
When to Use Insertion Sort
Utilizing an insertion sort algorithm can be an effective solution under certain conditions:
Benefits of the insertion sort algorithm include its low overhead and simplicity. When a pre-sorted or partially-sorted input set is expected or known, performance of the insertion sort algorithm can be significantly better than many alternatives, including divide and conquer algorithms such as merge sort, heap sort, even QuickSort.
When Not to Use an Insertion Sort Algorithm
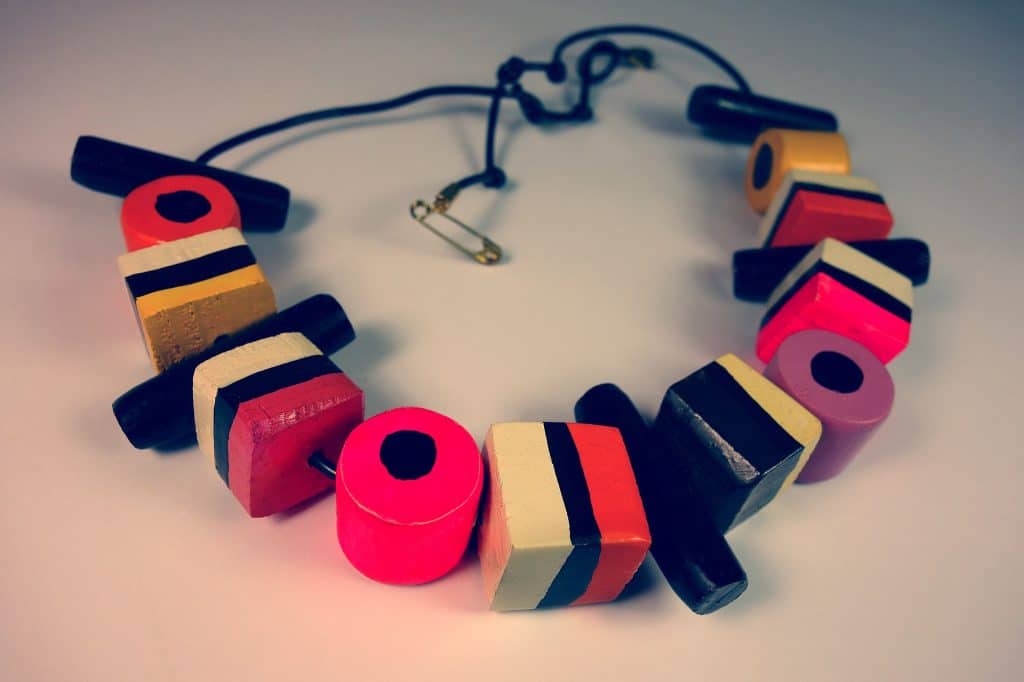
In many instances, the size of the input set to your sort algorithm is unpredictable, or you may even be aware that the volume of data will be large. In such use cases, insertion sort will not be a good choice to solve your sort requirements.
With average and worst-case scenarios (refer to Big O Notation later in this article), alternatives such as merge sort and heap sort will provide better performance.
Insertion sort is not your best choice when concerned with:
Making the Best Choice for Your Sorting Algorithms
Mathematicians and computer scientists have developed a set of guidelines termed Big O Notation, which provides guidelines for the efficiency of different sorting algorithms based on critical factors:
Binary insertion sort
These algorithm variations have even been compiled into a “cheat sheet” that provides a quick reference to these factors, including performance in best, average, and worst case scenarios.For an insertion sort algorithm, worst case conditions occur when the input set is in reverse order, with best case being where the input set is already sorted.
Additional information, including tutorials on Big O Notation can be found on YouTube and on multiple websites.
It pays to do a little research before making your final choice of sort algorithm solutions. There are divide and conquer algorithms that determine the size of the input set first, and automatically switch to another alternative such as selection sort or insertion sort to process small arrays more efficiently.
Sorting algorithms that are right for your application will depend on the volume of data to be sorted, the condition of the data itself (duplicate values, pre-sorting, etc.), space requirements, and even the programming language in use (not all sorting techniques are supported by every language).