What Is the C++ Vector Function?
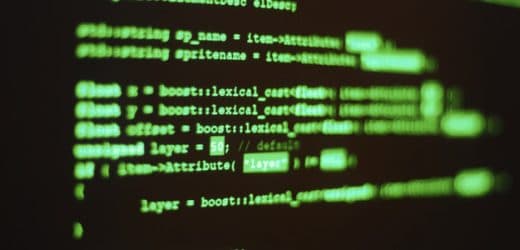
Spend some time talking to developers, and each has their favorite language. Many are happy to talk about SQL, Ruby/Rails, Swift, or Java as their favorite programming language. Ask them what their second favorite language is and the answer is almost always C++.
Many developers insist C++ is the best language for managing complex operations. It delivers very reliable performance for intricate operations, with a small programming footprint and low-energy.
But it’s also a very complex and demanding language. It’s not a language for easy work, simple apps, or junior developers. The power of C++ requires programming skill and developer acumen and experience.
The C++ Vector is a powerful bit of functionality in the developer’s toolbox. We’ll look at how the C++ vector function works, how it can be used.
Let’s get started.
The History of C++
C++ is an older language, first developed in 1979 by Bjarne Stroustrup. Stroustrup was working with the Simula 67 language at the time, a language widely thought to be the first object-oriented programming language. He felt Simula 67, while a useful language, was too slow for general programming.
Stroustrup began developing a new language known as “C with Classes” to add the object-oriented programming to the C language. C was a popular language, widely respected for portability, speed and functionality. Stroustrup added functions to C that included classes, inheritance, inlining, and default function arguments.
In 1983, the ++ was added to C to denote the ++operator for incrementing a variable. This helped identify the strength of the language. Additional features were added to C++, including function overloading, references with the & symbol, virtual functions, and the const keyword.
Since then, C++ has become a useful language for general-purpose programming. Many popular programs are written in C++, including Adobe applications, programs in Microsoft, and parts of the Mac OS. Applications that require high-performance, eking out every bit of performance from a CPU, also rely on C++. This includes audio/video processing, 3D rendering, and fast, or twitch, game development.
This is why many mainstream games and gaming companies rely on C++. Popular
apps like Facebook and Amazon also use C++. C++ also eliminates resources when released to increase performance, making it the right choice for industries like finance, high-performance manufacturing, real-time systems and transportation.
Let’s look at how vectors work in C++.
What is a C++ Vector?
A vector in C++ acts as a data storage unit, sequence container, and a dynamic array. As elements are added to the array, the vector will automatically resize itself to accommodate the new element. When elements are removed, the vector will adjust.
A typical array in C++ is fixed in size and contains sequential elements all of the same type. They are used to store data. Arrays always use connected memory locations. The
lowest address is assigned to the first element, and highest address is assigned to the last element. Since the array is of a fixed size, the number of elements must be determined when the array is created.
Vectors work like an array, but don’t have a fixed size. The vector will adjust as needed
to accommodate data as it is added and removed. In practice, a vector will use more memory than a similarly-sized array to accommodate expected growth, but it does efficiently and dynamically grow to incorporate new data as needed.
Properties of a C++ Vector
A vector container in C++ has certain properties. These include:
- Linear sequence: Elements in a vector in C++ have a strict linear sequence. Each element in the sequence can be accessed by their position in the sequence.
- Dynamic access: A vector permits direct access to any element in the container. The vector enables rapid addition and removal of elements at the end of a sequence.
- Allocator-aware: The vector uses allocators to manage storage needs. An allocator is part of the C++ Standard Library and manages requests for the allocation and deallocation of memory for data containers.
Implementation of a C++ Vector
The types of elements that can be stored by the vector depend on how the vector is initialized. Remember, all elements in a vector must be of the same type. Vectors can store string, float, and int elements.
Selecting an allocator object when implementing a vector will also define the storage allocation model. By default, an allocator class template is used. This assigns the simplest memory allocation model. This model is value-independent.
Iterators for C++ Vector
An iterator is a fundamental part of the C++ Standard Template Library. Iterators are used to access the data stored in the vector. They point to the specific element within
the data storage unit or container.
Let’s look at some common iterators for vectors in C++:
- begin: Using the begin iterator will return the element in the beginning of the sequence in the vector.
- end: The end iterator will return the element at the end of the dynamic array, or the theoretical element that follows the last element.
- rbegin: This will return the reverse iterator to the reverse beginning of the vector. This will move from the last element to the first element.
- rend: This will return the reverse iterator. It points to the theoretical element immediately before the first element in the sequence. It is the reverse end of
the vector. - cbegin: Using the cbegin iterator will return the const_iterator to the beginning. A const_iterator can be used for accessing the elements in the container and cannot be used to modify the data.
- cend: Using the cend iterator will return the const_iterator to the end. A const_iterator can be used for accessing the elements in the container and
cannot be used to modify the data. - crbegin: This will return a const_reverse_iterator to the reverse beginning of the dynamic array.
- crend: This will return a const_reverse_iterator to the reverse end of the dynamic array.
C++ Modifiers for a Vector
C++ Modifiers are another important feature in the programming language. When char, int, and double data types have modifiers preceding them, the base type can be modified. Modifiers can be used to adjust the type to fit programming situations.
In general, a vector is more efficient than other dynamic sequence containers in C++, including deques, lists, and forward_lists. They are suited to adding or removing elements from the end of the sequence. They are less efficient at inserting or removing
elements within the sequence.
C++ Modifiers that can be used on elements in a vector include:
- assign: The assign modifier will assign data or content to the vector, adding the element to the container.
- push_back: This modifier will add the element it modifies to the end of the sequence in the container.
- pop_back: Use the pop_back modifier to delete the last element in the sequence, shrinking the vector.
- insert: The insert modifier will insert the specific modified elements to the sequence.
- erase: The erase modifier is used to delete or eliminate specific elements in the sequence.
- swap: Use the swap modifier to move content inside the sequence.
- clear: The clear modifier will remove, or clear, content inside a sequence. It uses the location within the sequence, rather than the element.
- emplace: Use emplace to construct, or build, an element and the insert it within the sequence.
- emplace_back: The emplace_back modifier is used to construct an element and insert it at the end of the sequence.
Calculating Vector Capacity in C++
The capacity function is a built-in function in C++ which can be used to calculate the storage assigned to the vector. The capacity is expressed as the number of elements.
Keep in mind the capacity that is returned is not necessarily equal to the number of elements in the vector. Extra space will often be assigned to a vector to accommodate growth. This way, the system won’t need to reallocate memory every time an element is added to the sequence.
Capacity also doesn’t limit the size of the vector. Adding elements will automatically expand the size of the dynamic array as needed. A limit to the size of a vector is a property of the member max_size.
Functions on capacity for vectors in C++ include:
- size(): Calculates the number of elements in the vector.
- max_size(): Calculates the maximum number of elements a vector can contain.
- capacity(): Calculates the storage space currently assigned, or allocated, to the vector. This is expressed as the number of elements.
- resize(): This can be used to resize the vector to contain a specific number (“g”) of elements.
- empty(): Determine if a container, or vector, is empty of all elements.
- shrink_to_fit(): Used to limit the capacity of a vector. The container will be shrunk, and all elements beyond the capacity will be destroyed.
- reserve(): A request to assign enough vector capacity to contain at least a specific number of elements.
A Final Word on Vectors in C++
Vectors, or dynamic arrays, are a powerful tool for storing and accessing information in C++. As more and more companies are using C++ to store and use data, demand for programmers knowledgeable in C++ will only increase.
.
What do you think?