Generating Random Numbers with Java Random
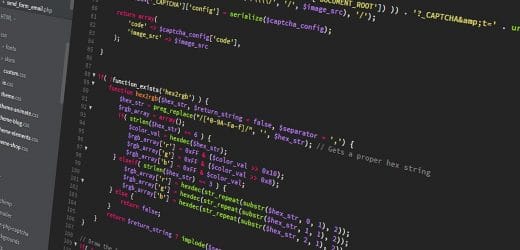
Java has a number of functions that can be leveraged by the programmer to create desirable effects. One function, in particular, helps with procedural generation, game development, and a variety of other applications.
Random number generation in Java is a powerful feature that lets the programmer generate random numbers. Objects of the Random Class come with a variety of additional methods that can return a variety of output.
There are many reasons a developer would want to use random number generation in Java. Game development, dice rolling, and procedural generation are all practical applications of the java random number generation.
We will walk you through how to generate random numbers with java random, practical applications of the Random class, technical info, and some common mistakes programmers run into when using this class.
How to Generate Random Numbers with Java Random
To better explain how to generate random numbers in Java, we will break up the information into the following 6 sections:
- Overview
- Import
- Instantiation
- Constructor
- Methods
- Example
OVERVIEW
The Java Random class is a powerful tool that allows Java developers to generate random numbers. These numbers can be returned as regular integers, floats, or doubles. The output that a programmer desires will be based on their specific needs.
It is important to know that Java Random is primarily a class. To use this class, the programmer must create an object to represent an instance of the Java Random class. This created object will have access to the constructor and various methods provided by the Java Random class.
It is also important to note that the Java Random class does not provide true random numbers, but it provides pseudorandom numbers. For example, if two Java Random classes are instantiated with the same seed, then they will both produce the same pseudorandom number.
Because these numbers are produced based on an algorithm, and can be replicated, they are not truly random.
IMPORT
In order to generate random numbers with Java, you must first import the Random class from the package java.util. The code for importing the Random class is as follows:
- import java.util.Random
This will import the Random class and make it available for use.
Instantiation
After you import the Random class, you must then instantiate the class with a valid constructor. In order to instantiate the Random class, you must create a Random object.
To do this, you can write the following code under your public static void main method:
- Random randomNum = new Random();
This code will instantiate an instance of the Random class in the Java Object randomNum. Any time you want to use the Random class, you can call its methods through randomNum.
CONSTRUCTOR
There are two different formats in which the Java Random class can be instantiated. These formats are defined by the constructor, the code that goes into the parenthesis when we instantiate the Random class.
- Random randomNum = new Random(constructor)
There two constructors that exist for the Random class are as follows:
- Random()
- Random(long seed)
The first constructor, Random() simply creates a new random number generator.
On the other hand, the second constructor, Random(long seed), generates a new random number generator based on a long seed. A long seed is a number with the datatype long. When you seed a random number generator, you should receive the same random numbers.
This may sound confusing, but the same numbers are generated because seeded random numbers are generated by a pseudorandom number generator. These generators use algorithms to generate sequences of numbers. If you use the same seed in two different Random classes, you should receive the same random numbers.
METHODS
The methods provided by the Random class is where the random number generation actually happens. We will cover the following common number generation methods:
- nextInt()
- nextFloat()
- nextDouble()
The nextInt() function returns a pseudorandom number that will exist as an integer object type. Integer values are common, making this function particularly useful if you are primarily dealing with integer values.
The method nextInt() can also take a parameter that limits how high the value of the integer returned can be. For example, say you have a Random class instantiated as randomNumGen. The method call randomNumGen.nextInt(10) would return an int value in-between the value of 0 and 9.
The value you set is exclusive, meaning it won’t actually be a number included in potential integers returned. If you wanted a random int generated between 1 and 10, you would have to write randomNumGen.nextInt(11).
The nextFloat() method returns a float value between 0.0 and 1.0. This method is useful for generating precise numbers that can be manipulated in a variety of fashions.
The nextDouble() method returns a double value between 0.0 and 1.0. This method is useful if you want to generate a decimal value of up to 15 to 16 points. Double values are more precise than float values.
Example
Image via Pexels
Now that you understand how the Random class works, is instantiated, constructed, and how its methods are called, you should be able to follow how the random number generation works in application.
In the following code, we detail a simple program that uses the Random class and the nextInt() method.
import java.util.Scanner;
import java.util.Random;
class RandomNumberGenerator
{
public static void main(String[] args){
int maxRange;
//create a scanner to output print
Scanner sc = new Scanner(system.in);
//create a random number generator
Random randNumGen = new Random();
System.out.printlin(“Enter a maximum range: ”);
maxRange = sc.nextInt();
for(int i = 0; i < 9; i++ ){
System.out.println(randNumGen.nextInt(maxRange));
}
}
}
When you run this code, you will be prompted to enter a random number with a maximum range. After entering the number, you will receive 10 outputted numbers from the random number generator.
Practical Applications of the Random Number Generator
Image via Pexels
The Java random function has a number of useful practical applications. The following applications of the explode function help make it a powerful tool for programmers:
- Simple RPG Critical Hit Mechanic
- Dice Rolling
- Procedural Generation
Simple RPG Critical Hit Mechanic
The Java random number generator is an excellent tool to use for creating simple RPG combat. RPG combat is heavily based on chance influenced by numbers. One specific RPG modifier, critical hit chance, is the chance that a player has to do critical damage.
You can use the random number generator to generate a number in a range from 0 – 100. If your character has a 50% critical chance on their attacks, then you can use the random number generator to simulate this.
If you roll a number that is between the values of 0 and 50, then you score the critical hit, if your number is between 51 and 100 then you don’t score the critical hit. You can practice different critical hit thresholds, and use the random number generator to model.
Dice Rolling
You could use the random number generator to simulate a dice roll. You could simulate a simple 6 sided die for simple games. You could also simulate a d20 die which you could use to simulate dice roles for roleplaying games.
Procedural Generation
Procedural Generation is a technique in which portions of a world are generated based on a set of specifications. This technique relies heavily on algorithms and random number generation. For example, you could use a random number generator to load in different gameplay levels for a player.
You could also use Procedural Generation to create different item names, stats, and locations. Items are procedurally generated in this fashion in games like Diablo III and Destiny 2.
Technical Information
One of the most important elements to remember about random number generation with Java is that the random number generator is not truly random. It is based on a linear congruential formula that relies on a 48-bit seed to generate random values.
Common Mistakes
There are a few key mistakes that programmers tend to make when using the random numbers class in Java. One of the first mistakes is believing that the numbers generated by Java random are truly random.
One of the most common mistakes programmers have is that they simply cannot use the Random class. You must remember to import the Random class when you want to use it in an instance. If you don’t include the Random class in your java file, then you won’t have access to it.
Generating Random Numbers with Java Is Easy
Generating random numbers with Java is a relatively straightforward process. The Java Random class is powerful and has a number of real-world practical applications.
If you have an interest in roleplaying games, we recommend you create your own dice rolling program to use with a role-playing board game. You could even create new types of dice simulations with the Java Random class.
Featured image source: Pexels
What do you think?