What Is the PHP Explode Function?
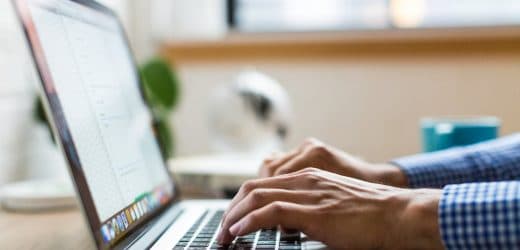
PHP has many functions that make programming large applications easier. One of those functions is the PHP Explode Function. The Explode function allows users to break a string up into an array.
There are many reasons why a developer would want to break a string up into an array. Different applications, data patterns, and problems might need you to manipulate a string. The PHP explode function allows you to parse through a string in an easy array format.
We will walk you through how the PHP Explode function works, applications for the function, technical info, and some common mistakes programmers run into when using this function.
How the PHP Explode Function Works
The Explode function requires 2 necessary parameters and 1 optional parameter. Those parameters are as follows:
- Separator
- String
- Limit (optional)
We will break down what each of these parameters is, and why they are necessary to produce valid output from the explode() function.
SEPARATOR
The separator specifies where exactly the function should break up the string. For example, say you have a string that reads as follows:
- “Shek Wes has so many flows.”
You could decide to make the separator a space (“ “). If we use “ “ as a separator then the created string would be represented in an array. [Shek, Wes, has, so, many, flows.]. Note the period character behind flows is still added to flows in the array since there is no space separating the s from the period.
It is also important to note that separator cannot be an empty string (“”).
STRING
Image via Pexels
The string parameter is the string that you input. This is a required parameter. If you do not add this parameter, the PHP explode function will not work.
LIMIT
The limit is an optional parameter that defines the number of elements that will be returned in the array. There are 3 different types of possible values. Those values are as follows:
- Greater than 0
- Less than 0
- 0
Limits with a value that is greater than 0 will return an array where the maximum number of elements is determined by the value the programmer inputs for the limit. For example, a string that reads, “Luke I am your father” with a limit of 3 will return the array [“Luke”, “I”, “am your father”]. Notice that the final element in the array contains more words.
In another example, let’s say the string you want to explode reads, “Hello World!”. If you input a limit of 1, then the array returned will be [“Hello World!”].
Inputting a limit that is less than 0 will return an array that removes the number of elements defined by the limit from the back of the array. A string that reads, “Luke I am your father” with a limit of -1 will return [“Luke”, “I”, “am”, “your”]. Think of this function as simply removing elements from the end of your array.
A Limit that is defined as 0 will return an array with 1 element. A string that reads, “Luke I am your father” with a limit of 0 will return [Luke I am your father].
PUTTING IT ALL TOGETHER
Now that you know the parameters necessary to successfully use the explode function. We will walk through a full example with you.
$phrase = “Be formless, shapeless, like water.”
$wordFragments = explode(“ “, $phrase);
for ($i = 0; $i < count($phrase); $i++){
echo “Word $i = $phrase[$i] <br />”;
}
This code takes the phrase “Be formless, shapeless, like water” and prints out the following list:
- Word 0 = Be
- Word 1 = formless
- Word 2 = shapeless,
- Word 3 = like
- Word 4 = water.
If we change $wordFragments and change $wordFragments to $wordFragments = explode(“,”, $phrase); then the following output should occur:
- Word 0 = Be formless
- Word 1 = shapeless
- Word 2 = like water
Applications of the PHP Explode function
Image via Pexels
The PHP explode function has a number of useful practical applications. The following applications of the explode function help make it a powerful tool for programmers:
- Technical Interview
- Creating JSON Simply
TECHNICAL INTERVIEW
Data structures are some of the most important concepts that folks need to be ready for when preparing for a technical interview. Arrays are common data structures that are used in these interviews. Similarly, there are many problems that focus on string manipulation.
The PHP Explode function comes in handy by providing you a way to break up a string and convert it into an array. This data structure makes it easy to parse out certain words.
You might be tasked with counting the number of times a word occurs in a paragraph. To count the number of times a word appears in a paragraph, you can break the paragraph up with an empty string (“ “) as your separator.
Once you explode the paragraph string, you can count the number of times a word appears. You can do this by parsing the exploded paragraph with a for loop. Count the occurrences of each word, and make sure you pay attention to punctuation as periods, commas, and dashes will still be added to words.
CREATING SIMPLE JSON
JSON is a data structure that is commonly used on the web. JSON is useful because it is a data structure that is easy to read and write. If you are using PHP to parse and encode JSON, then the explode function will definitely be valuable for you.
The JSON encode function requires an array and will encode that array and convert it to a JSON object. The PHP explode function comes in handy when you want to create an array with specific value from a string. Using PHP explode gives you an easy way to break apart a string based on the values you actually need.
PARSING A LIST
Another practical application of the explode() function is parsing a list. In an application, say you are asking users to input the food items they are interested in. It is easier for the user to enter these items into 1 textbox instead of many.
It is then on you as the programmer to read this input and parse it. The explode() function would make parsing a list trivial. You also have the added benefit of being able to search through the array simply. With a for loop, you can pull each individual string out of the array generated by the explode function.
After you pull each individual string from the array, you can manipulate the data in whichever way you see fit. Parsing a list is a common application of the PHP explode() function.
Technical Information
W3 schools has an excellent changelog for when certain updates were made to PHP.
The PHP explode() function was added in the 4th iteration of PHP. The limit parameter was added in the 4.0.1 PHP update. Negative limit support was added in PHP 5.0.1.
Common Mistakes
There are a number of programmers who have gathered their collective knowledge on Stack Overflow and PHP.net. On these sites, folks share common mistakes and issues they face with the PHP explode() function.
One of the common mistakes programmers run into is supplying an empty string to the PHP explode() function. If you try to explode() an empty string, then the function will return an array with 1 element. This element will have a key, 0, and an empty string as that key’s value.
Another mistake programmers encounter is trying to explode a string with quotes inside of it. There are multiple ways to get around this problem. You could decide to split the string on the quotes and then explode the string again.
It is important to remember that the explode() function relies on the separator to parse the string. You will have to handle errors and further process output based on how you want to use the data. Take advantage of for loops to check that the data in your array is what you expected.
Creating test cases will help you solve many of the programming issues you encounter while using the explode() function.
The PHP Explode Function Is a Powerful String Parser
The PHP explode() function is a powerful function that you can use to parse strings and convert them into an array. Because an array is such a useful data structure, there are many instances where the PHP explode() function can help make your job easier.
Remember to create ways to test your code! This will ensure you properly use the PHP explode() function. Make use of resources like this site and coding forums like StackOverflow if you run into issues with the explode() function.
Featured image source: Pexels
What do you think?