C++ String
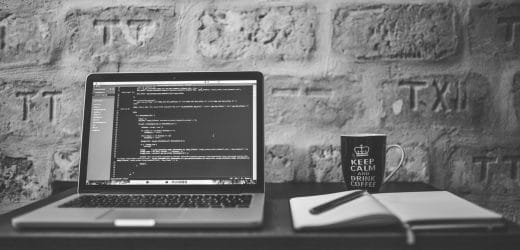
C++ is one of the most popular programming languages to learn and is known as one of the fastest-running programs for applications developed with the language.
This is one of the attributes that makes C++ a preferred architecture for writing game applications, with its combination of power and speed.
In a technology-based world where such languages as Python and JavaScript have a growing segment of the application development market, C++ remains strong for its advantages in powering such applications as:
- Financial systems
- Computer gaming development
- Search engines such as Google, where performance is critical
- Social media applications, including much of Facebook
One other attribute of C++ is it’s very complex and is not considered by most technicians to be a great first language to learn, nor an easy language to become proficient with.
One function included in the C++ language is that of the “string” function and class.
What Is the C++ String Function?
There are two types of string representation supported and provided by C++:
C-style string representation – since C++ is largely an evolution of the C language (though now quite different), C++ still retains the basic functionality of C-style string handling.
A string is a one-dimensional array that is terminated by a null value (‘\0’). This being the case, the size of the array is always the length of the characters in the string, plus one position for the null character.
In C++, a string is an object within the “string” class and is defined as such with a <string> header.
Constructors may be called to define and create a string object:
- string str1; creates a null string with a zero length using the default constructor
- string str3(str2); utilizes an explicit constructor to create and initialize the new string object
Comparison Table
[amazon box=”1520811055,1983229288,1484233654,B01HXJGKHM,B001FWIJFU,0596004192″ template=”table”]
Coding a String Function in C and C++
C offers a variety of functions for handling and manipulating strings terminated by nulls
- strcpy(str1,str2); – copies string str2 into string str1
- strcat(str1,str2); – concatenates str1 and str2, with str2 placed on the end of str1
- strlen(str1); – returns the length of the specified string (str1)
- strcmp(str1,str2); – compares two strings – if the two strings are equal, a value of 0 is returned. If the str1 is less than str2, a value < 0 is returned. If str1 is greater than str2, the returned value is > 0.
- Strchr(str1,ch); – value returned is a pointer to the first occurrence of the character specified in the ch value
- Strstr(str1,str2) – returns a pointer to the first occurrence of the string specified as str2 in string str1
Each of these functions certainly has effective capabilities and use in working with character strings.
C++ includes support for these C string functions, but also takes string capabilities to another level, with the string class designation.
[amazon link=”1520811055″ title=”C++ Programming Language” /]
[amazon box=”1520811055″]
[amazon link=”1983229288″ title=”C++ for Beginners” /]
[amazon box=”1983229288″]
Defining a C++ String
There are several ways to define a string in C++, including the C character method:
- char str[] = “test”; – this example defines a 5-character string, consisting of the word “test” plus a null character to terminate the string, which is added automatically, to verify the end of the string
- char str[5] = “test”; – this syntax produces the same results
- char str[] = {‘t’, ‘e’, ‘s’, ‘t’, ‘\0’}; – same results again, but the null character has been included in the coding
When specifying a string, you can provide a string size that may not always contain that specific number of characters. For example, you may want to allow a string of 50 characters for someone to key an address, but the actual string entered could be much less, as in:
char str[50] = “123 Main Street”;
A simple program to illustrate the use of a string to accept data from a user at the keyboard:
#include <iostream>
using namespace std;
int main()
{
char str[50];
cout << "Enter your address: ";
cin >> str;
cout << "You said: " << str << endl;
cout << "\nEnter another line: ";
cin >> str;
cout << "You said: "<<str<<endl;
return 0;
}
Putting the C++ string class to use, you have many alternative ways to utilize string
functions and methods.
String attributes are easily determined for C++ string objects using methods such as:
length() or size() – methods return the length in characters of the string
C++ Operator Overloading
Operator overloading is a C++ function facilitated by the function of the string class named operator[]. This implements the operator overloading feature which enables the use of subscripting with a string. Subscripting allows you to access individual characters within a string, rather than the entire content.
Example: cout << str1[5] << end1;
the subscript (in brackets) will be passed to the operator member function, and the character in that position of the string will be returned.
[amazon link=”1484233654″ title=”Beginning C++17″ /]
[amazon box=”1484233654″]
[amazon link=”B01HXJGKHM” title=”Beginning C++” /]
[amazon box=”B01HXJGKHM”]
Additional C++ String Functions
Comparison
Strings can be compared, both to other strings, or to specific values:
- if (str1 > str2) – comparing the values of two strings
- if (“large” == str2) – comparing to find a size in an item description, for example
- if (str3 != cstring) – comparing the C++ string to a C string that is an array
These comparison functions also utilize the operator overloading method
Manipulation
Content of C++ strings can also be manipulated through the assignment of other strings or even by the use of a C character literal value, for example:
Assuming this string content
string s1 = "first string";
string s2 = "second string";
char s3[20] = "one more string";
This code would have the following effects:
s1 = s2; s1 changed to "second string"
s1 = s3; s1 changed to "one more string"
s1 = "final string"; s1 changed to "final string"
Here again, operator overloading makes it happen
I/O of String Content
Input and output can be facilitated through the implementation of the stream extraction operator >> that will read data into the C++ string object, and the insertion operator << for printing or displaying the string.
Concatenating C++ strings
The C++ operator “+” can be utilized to concatenate a combination of multiple C++ strings, string literals, and C strings together, in any order you need. When concatenation is performed, the result is a C++ string that can be passed to a function for use as a C++ string object, assigned to a new C++ string object, printed, or whatever you may choose to do with the concatenated results.
string str1 = "Lucy";
string s2 = " finally ";
char s3[10] = "home";
s1 = s1 + ", I’m " + s2 + s3; // s1 now contains "Lucy, I’m finally home"
Passing and returning string content
By default, C++ string objects are passed and returned by value. The effective result is that a new copy/version of the string object is created.
Passing a string utilizing a reference will save memory and improve performance since the copy constructor does not need to be called when the string is passed in this way.
String Type Conversion
There are certain instances where you may have one type of string but need to use a function or method that requires a different type. Fortunately, there are simple ways in C++ to convert one string type to another type.
One easy way to accomplish string type conversion is by declaring the C++ string object you need, then passing the string literal or C string you have as a constructor
argument.
If the opposite condition is true, where you need to convert a C++ string object to a C string, that can be accommodated, as well. C++ string class provides a method called c_str()
that returns a pointer to a char
constant, which is the first character of a C string that is equivalent to the contents of the C++ string. This results in returning a C string. This C string cannot be modified, but you can still use it for printing, copying, or other purposes.
Example:
char str1[20];
string str2 = "C++ string";
strcpy(str1, str2.c_str()); This will copy the C string "C++ string" into the array str1
Splicing or Erasure String Content
A very useful function for modifying string content is the erase() function.
This function allows you to examine and modify C++ strings to either remove or add content in a string. Erase() function syntax is similar to substr, taking a position and a
character count and removing that many characters beginning at that position (remember this is relative to zero).
string erase_sample = "remove 123";
my_erase_sample(7, 3); erases 123 from the string
To delete an entire string:
str.erase(0, str.length());
Another feature is the capability to splice one string into another. The insert member function takes a position and a string and inserts the given characters at the specified position:
string insert_sample = "145";
insert_sample.insert(1, "23"); the string insert_sample will now contain “12345”
[amazon link=”B001FWIJFU” title=”Secure Coding in C and C++” /]
[amazon box=”B001FWIJFU”]
[amazon link=”0596004192″ title=”Practical C++ Programming” /]
[amazon box=”0596004192″]
Using the C++ String Function
When writing C++ applications, use true C++ strings whenever possible, to avoid the use of alternatives such as arrays. Although this may not always be possible, it’s beneficial to avoid using C strings. There are some instances where C strings will be required, at least in current versions of C++:
- Command line content is passed into
main()
as C strings - Some very useful C string library functions to date have no equivalent in the
C++string
class - If you need to interact with other applications that may not incorporate C++ string support, you may be required to utilize C strings or even constant character strings.
Regardless of the strings that are passed to your application, within the confines of your own programming, it is normally best to leverage the benefits of the C++ string class to manage such information efficiently.
As with most development environments today, there are many resources available to extend your knowledge of C++ strings and how to use them efficiently.
.
What do you think?