Java Tips: How to Convert String to Int
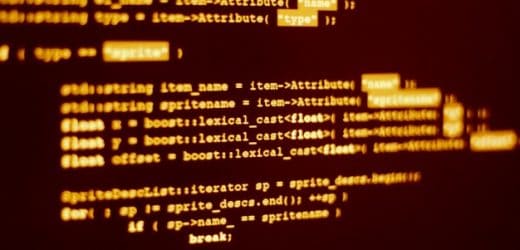
Java gives you all the tools you need to create robust, high-performing websites and applications for a variety of devices, from enterprise mainframes, to tablets, to smartphones and other mobile devices.
Building such applications, of course, includes accepting data from users in the form of keyed or uploaded data to be validated and processed by your programs. Data comes in many forms that include characters, numbers, and symbols.
Java supports multiple forms of data, among them are string and integer objects. One of the data objects most commonly utilized for accepting and working with data is the basic form – a string object.
But to utilize string data for specific purposes such as performing calculations or storing in database fields that have numeric properties, the string content must be transformed to an integer object. Attempting to perform an arithmetic function on a string object will get you in trouble with an error occurring immediately. You must first transform the numeric value in the string to an acceptable data type.
That being the requirement, the question is – how to convert string to int object content.
Java Has Options to Convert String to Int
Regardless of how new you are to Java programming, it’s quite likely that your first experience will require the function of performing numeric operations on data that is initiated in a string object form. Knowing how to make the conversion to fields you can treat as numbers will be critical to writing a successful application.
Java provides multiple ways to transform string content into integer objects. There are multiple types of integer objects, to make the subject more confusing. Java provides four primitive integer types that store whole numbers of varying sizes:
- Byte – 1-byte integer that can contain a range of values from -128 to 127
- Short – 2-byte integer with a range between -32,768 to 32,767
- Int – 4-byte integer with range between -2 billion and 2 billion
- Long – 8-byte integer with range between -4,000 trillion to 4,000 trillion
The most common selection for an integer object is int, due to its range for most numeric values
When you know a small value will be used for a particular function, byte or short can provide an efficient use of memory. Long is an exception, but may be applicable for some applications.
These data types indicate to your program not only that the field is a numerical variable, but also the range of values it can contain, and the operations the variable can be have performed on it.
The issue is that it’s often the case that you begin with a string data type, which must be transformed to an integer type to perform numeric functions. Two options are available to you in Java:
Option 1 – Integer.parseInt()
A simple example:
string number = “50”;
int result = Integer.parseInt(number);
the result will be a primitive integer with a value of 50
The parseInt syntax is:
parseInt(string s, int radix)
s = the string to be converted to an integer – the sign needed for the integer may also be specified
radix – the radix to be used during string parsing
You can also perform a simple conversion from string to an integer with this function:
parseLong() – parses the string to Long integer data type
Option 2 – Integer.valueOf()
String number = “50”;
Integer result = Integer.valueOf(number);
The result is a new integer object with a value of 50
Integer.valueOf syntax is:
Integer valueOf(int a)
This results in a new integer representing the parameter a
The same syntax applies to variations of the valueOf and parse functions for other data types:
- Short.valueOf
- Long.valueOf
- parseShort
- parseLong
The primary difference between parseInt and valueOf functions is that parseInt returns a primitive int, but valueOf returns a new integer object. In fact, valueOf actually uses the parseInt function behind the scenes.
With either of the options, if the string contains a value that cannot be parsed to a valid integer, the code will throw a NumberFormatException error.
- String1 = “Hello world 12345”; would result in an exception
- String1 = “12345”; will be parsed without and exception being thrown
Due to the potential for any string containing a value that cannot legitimately be parsed, your code should always either:
- Validate the string content prior to attempting to convert a string to int.
- Handle the NumberFormatException within your program.
- Strip any invalid characters from the string before parsing (possible logging a warning that data has been omitted).
Selecting the Right Type of Java Int Object
With the multiple options available to you for numeric variables, you need to be cautious in the object type selected for individual functions.
Values that you know will be small such as counting entries on a web page screen may easily be contained within a byte or short data type. Int type will handle most typical application values, but if you may encounter large values, long data type can be your best option.
If you opt for a smaller capacity data type but encounter larger values, you could conceivably overflow the capacity of your integer object.
Examples of How to Convert String to Int?
You have the basics now for functions that allow you to convert strings to numeric variables using integers of different types, so let’s look at some additional examples to illustrate the coding.
Additional example1 using Integer.parseInt()
String strTest1 = "500";
int iTest = Integer.parseInt(strTest1);
System.out.println("Original String:"+ strTest1);
System.out.println("Converted to Int:" + iTest);
This routine will result in the original string of “500” converting to the integer object iTest, displaying both the original string and new integer
Additional example2 using Integer.parseInt()
String ostr="123";
int inum = 456;
/* convert the string to int value
* ,making the value of inum2 123 after
* conversion
*/
int inum2 = Integer.parseInt(ostr);
int sum = inum+inum2;
System.out.println("Result is: "+sum);
Additional example1 using Integer.valueOf()
String strTest1 = "1000";
int iTest = Integer.valueOf(strTest1);
System.out.println("Original String:"+ strTest1);
System.out.println("Converted to Int:" + iTest)
Additional example2 using Integer.valueOf()
String istr="-234";
//int variable
int inum1 = 110;
/* Converting String to int in
* the value of variable inum2 negative after
* conversion
*/
int inum2 = Integer.valueOf(istr);
//Adding up inum1 and inum2
int sumo = inum1+inum2;
//displaying results of sumo
System.out.println("Result is: "+sumo);
Final results of the valueOf and parseInt methods of converting string data to int objects are the same, aside from the type of the object returned.
In Summary
In order to perform any numeric functions such as arithmetic on an object in Java, the information must be in contained in a valid numeric object type. Even if a string contains all numeric data, an error will occur if you try and use it for any type of calculation.
Source: Freepik.com
You can perform this conversion from string to int with two Java coding methods:
- Integer.valueOf(string)
- Integer.parseInt(string)
The difference between the two:
- parseInt returns a primitive int value
- valueOf returns an Integer class object with the converted value
- valueOf may optionally contain a negative value, provided by a minus sign
Regardless of the method you choose in your Java development efforts, the primary considerations to keep in mind are:
- Be sure the int object you define from the string conversion has the capacity you can conceivably extract from the string – if in doubt, use a larger data type.
- Select an integer type that will allow all functions and values you anticipate, such as negative values or floating point operations.
- Verify the string contains valid data that can be parsed successfully, or at least include code to detect any exceptions thrown from a conversion error.
More Details for Java Conversion String to Int
There are many great resources available to you as a Java developer, to gain additional knowledge of how to convert string to int values.
- Technical web sites dedicated to providing information and examples to technicians.
- YouTube video tutorials
- Hundreds (perhaps thousands) of great books on Java programming and coding tips.
Each of these options will provide an amazing array of how-to instructions on Java programming topics, with a variety of examples, drawing from the experience of seasoned Java developers.
What do you think?