How to Use ForEach in JavaScript
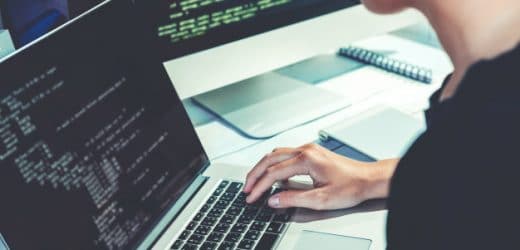
JavaScript is one of the most popular development tools or languages in use for creating websites and client-side applications today. Its capabilities and power are demonstrated through JavaScript’s presence in social media sites such as Facebook and Twitter, as well as adoption by powerful search engines that include Google.
As a JavaScript developer, you’re always on the lookout for tips, tools, and methodologies that can make your software engineering life easier, more productive, and more efficient.
Among these techniques is the appropriate use of JavaScript’s “foreach” method of handling arrays and controlling program flow. You probably are quite accustomed to using the “for” loop in your programming, but there are several nuances and scenarios where the foreach method may contribute to a more efficient way of coding for your application.
What is the ForEach Method?
Foreach is a coding method that controls the flow of logic when handling an array or some collection of sequential elements.
As with the for logic method, foreach in JavaScript is utilized to perform a certain set of logic or an operation on the input elements. Unlike a for loop, foreach utilizes no counter or iteration value to control the execution of the loop. Foreach essentially tells the program to perform the specified logic on every entry in the collection of elements.
By comparison, JavaScript’s for loop includes a combination of three parameters or statements, defining the execution of the logic to be executed through the loop code. For example:
- For (i = 0; i < 20; i++) { }
- i = 0 initializes the iteration to 0
- the loop will execute as long as the iteration index remains less than 20
- i++ will increment the iterator by 1
Logic coded between the brackets will be executed for each iteration of the loop. This basic functionality of loop processing is a powerful and useful cornerstone of JavaScript. It has been utilized for its versatility in creating iterative code by practically any developer who was to put fingers to a keyboard.
The trick when coding a for loop is knowing where to set the iteration value – setting it too low could result in fewer than all elements in the input array being successfully processed through the intended logic of the function.
ForEach operates in a different manner, giving you a method that can perform a function on every element in an array, map, or set.
The most common use of foreach method is to loop through a sequence of elements and perform the same logic for every element in the array or collection. There is no explicit counter for the number of items to be processed through the foreach loop – every element is processed.
Here is a simple illustration of JavaScript code utilizing a for loop vs. a foreach method:
var sample_array = ['a', 'b', 'c'];
for (var i=0; i<sample_array.length; i++) {
console.log(sample_array[i]);
//a b c
}
sample_array.forEach(function(current_value) {
console.log(current_value);
//a b c
});
ForEach Syntax in JavaScript
Foreach processing is supported in many languages besides JavaScript, although the details of the syntax vary from one language to another.
In JavaScript, the syntax for the foreach array method takes on the following syntax:
Array.forEach(function (currentValue, index, arr), thisValue)
Parameters include:
- Function – the function that will be executed for each array element – this is referred to in JavaScript as the callback function
- currentValue – this is the value of the current element
- index – (optional) array index of the current element
- arr – (optional) the array object the current element is part of
- thisValue – (optional) a value that you want to pass to the called function as its “this” value
AppendTo.com provides you with some very basic examples of coding foreach loops, which will give you a good foundation for the syntax and construction of this method.
How to Use ForEach in JavaScript
Now that you know what foreach does, how do you use it effectively?
You need to be aware of certain factors that apply to using foreach methods on arrays:
- forEach() sets the range of elements to be processed in the array before execution starts with the first element. This means that any elements that get added to the array after execution begins will not be processed by the foreach loop.
- If the content or values of array elements change after execution begins, the value recognized by the called function will be the value at the time that element is executed.
- If elements in an array have no values, the function is not executed.
- Also, elements that are deleted from the array before they are processed through the function will not be processed.
- Another caution – if elements that have already been processed are deleted, the process of shifting elements in an array will cause elements later in the array to be omitted from processing. To illustrate this effect, imagine an array that contains values of 10, 20, 30, and 40, and logic that is to summarize the values. If the second entry is deleted, while processing the array in a foreach loop, 30 and 40 will be shifted up. With the element containing 30 now in the second position, it will be skipped.
Foreach does not make a copy of the array before executing the loop, which could subsequently impact your results. This may not be an important factor in your application, but you must be aware of the condition.
Foreach method will not alter the content of the original array, although the function called may do so.
Each of these attributes of foreach processing is important to your programming design, especially when working with arrays that may be highly volatile in nature.
ForEach Browser Support
Nearly every popular browser supports the forEach JavaScript method, depending on the version in use:
- Internet Explorer (Ver. 9.0 and higher)
- Firefox (Ver. 1.5 and higher)
- Safari
- Opera
Browser compatibility makes use of the method nearly universally acceptable for your web page or other application development use.
Reasons to Use ForEach in JavaScript
There are reasons you may want to elect to use foreach methods in your code over other options including for loops:
- Readability – many developers view foreach loops as more comprehensive when reading code
- Lines of code may actually be reduced, with no need to define extra variables for controlling iteration
- Speaking of iteration, foreach reduces the likelihood of making mistakes with index handling, such as setting an index to 1 instead of 0, also known as “off-by-one” errors. The result can be a loop that includes one too few iterations, or one too many.
On the other side of the coin, there are instances where you may want to break out of a loop early, before processing all entries in an array. This could be where you’re searching for a certain name, and want to break out once you encounter it, rather than stepping through every element in the array. For loop has an easy method of breaking out of the iteration.
Breaking out of a ForEach Method in JavaScript
Can you break out of a foreach loop? Yes, there are methods available to break out of a foreach loop, such as forcing an exception based on detecting some condition.
Like many developers, you may view this as contrary to why you would utilize the foreach method over a standard for loop, since throwing an exception is not a “clean” programming methodology or best practice. This is stated very plainly in an MDN Documents page:
“There is no way to stop or break a forEach() loop other than by throwing an exception. If you need such behavior, the forEach() method is the wrong tool, use a plain loop instead. If you are testing the array elements for a predicate and need a boolean return value, you can use every() or some() instead.”
A full discussion on breaking out of foreach methods and alternatives is available online, as well.
Learning More About ForEach in JavaScript
There are other variations of foreach methods available to you, if processing an object other than an array:
NodeList.forEach() performs the same function as the array foreach method, but utilizes a NodeList as opposed to an array.
There are many examples and tutorials online for developing your skills in the use of foreach in JavaScript, and YouTube offers many videos with examples and discussions on the use of the method.
What do you think?