What Is the Getline C++ Function?
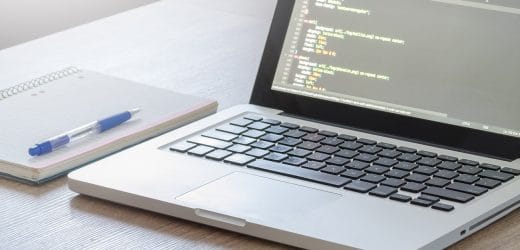
C++ is one of the most popular programming languages being used for application development today and has earned its popularity over a period of over 20 years (originally created circa 1979), with an initial standardized version released in 1998.
C++ offers developers many benefits:
- Object-oriented architecture – having such attributes as inheritance and polymorphism
- Platform agnostic – run your compiled C++ applications on anything from a desktop computer to a smartphone
- Low-level language – you work closely with platform characteristics such as memory management and device management
- Fast, efficient performance – C++ is a compiled language, as opposed to interpretive alternatives, providing performance similar to C applications, and generally out-performing C# programs
- Great for applications such as networking, creating operating systems, server-based functions, device drivers, and gaming
With an estimated population of C++ developers over 1.5 million strong worldwide, there are many sites, forums, and user groups available for training, tips, and collaboration.
C++ Getline Function – the Basics
Image via unsplash.com
When reading a data stream from any input source such as the keyboard or a data file, you have options for how you retrieve and manage that process. You can utilize the standard C++ cin function, or you may choose to use an alternative – the getline function.
What is the C++ getline function, and how does it work?
The getline function in C++ serves to extract characters or a stream of characters from a specified input stream, appending the stream to the string receiving the data until either a specified delimiter or new line is detected, or end of file is encountered.
Getline is a standard C++ library function and offers multiple format/syntax variations:
Format 1:
istream& getline (istream& is, string& str, char delim);
Parameters in this format are:
- is – an object in the istream class that provides information about the source to be read for input for the getline function
- str – the receiving string object where data is to be stored after the function reads from the stream
- delim – this parameter defines the delimiter character that tells the getline function to stop reading when it detects this character
Format 2:
istream& getline (istream& is, string& str);
This format is essentially the same as the first, with the exception that no delimiter character is provided. Getline function will recognize (‘n’) or new line character as the delimiter.
As an example using a delimiter character of “z”
istream& getline (istream& is, string& ‘z’);
In this example, if the ‘z’ character is found in the input stream, the extract will end, but the ‘z’ will not be included in the stream.
The basic function of getline is to extract data or characters from an input stream source, appending the information to the specified string object until new line or the specified delimiter is found. If the delimiter is detected, it is not passed to the string – it is discarded.
Note that the extract can stop for several reasons:
- The delimiter is encountered
- End of the source file stream is reached
- An error condition is detected
If data is received as a result of the getline function, it replaces any characters previously stored in the string object.
Conditions and Errors Returned by the Getline C++ Function
Image via unsplash.com
- Eofbit – this indicates that the end of the source stream characters has been reached
- Failbit – input data received by the function could not be properly interpreted as valid content of the type. In such instances, the internal data and parameters that existed before the call are preserved.
- Badbit – an error condition other than eofbit or failbit has occurred.
Most developers are quite familiar with the “cin” standard C++ default input method, especially when dealing with streams such as keyboard input. The result can sometimes be troublesome since the cin function may result in error conditions when it receives input content that it cannot process, resulting in a fail state from the function.
Until the fail condition can be corrected or cleared, the unprocessed information will remain on the input stream. To continue, either the input must be corrected for the function to proceed, or the fail state must be cleared and handled by your program.
Getline Is a Better Solution for Extracting Input
Image via unsplash.com
Getline is a more robust and error-tolerant method for reading an input stream for multiple reasons:
- Both numbers and strings can be read without resulting in a fail state
- When you want to read an entire file, getline can be easily embedded to read input through the file within a loop, extracting all content efficiently, and cleanly exiting the loop when end of file isreached.
- Getline is custom-made for processing through a delimited file since you’re able to provide the delimiter character to be recognized as your stop point.
Special Considerations Where the Getline C++ Function Shines
Image via unsplash.com
There are certain conditions where getline is your best choice for accepting a string from keyed input or when reading data from a file.
Blank Lines in Input Stream
Image via unsplash.com
Blank lines in an input stream can create a problem for your program since getline will interpret the newline character at the end of a blank line, sending the blank content to the output string.
Fortunately, by testing the length of the string, it becomes very easy to omit the blank entries, looping through the input stream for the next valid line, as in this code example:
while (str.length()==0 )
getline(cin, str);
This is an easy method of skipping stream content lines where the length of the string is 0 (bypassing blank lines).
Converting String Content
Image via unsplash.com
Using the string parameter in a getline function provides ease of applying the C++ converter functions to transform string-to-long-integer (stol), string-to-integer (stoi), string-to-double (stod), and string-to-float (stof) formats. Since the data has already been read into a string object, it is easily converted as needed by your application.
Coding and Use of the Getline C++ Function
Image via unsplash.com
There are some additional coding considerations that illustrate best practices or cautions against inefficient code:
When executing a getline function, memory will be allocated for the string to contain data from the input stream. If the function fails or otherwise encounters an error condition, the memory may not be freed for other use. In such cases, you should free the allocated memory. A good practice is simply to always free the allocated resource when finished with the function and data string.
Never just assume that your code worked flawlessly. C++ and its functions provide many options for discovering and identifying error conditions. Checking your status conditions when functions are executed will ensure that the expected results are realized and will also inform you of any data or program exceptions that need to be addressed.
Another best practice is to not mix types of input coding, such as using cin and getline in the same process flow. The reason – cin is well-known for encountering issues with input, mainly due to its lack of type tracking. This could result in a subsequent getline receiving empty results. It’s much more consistent and reliable to utilize the getline C++ function to provide better control of the data stream, error checking, and data manipulation.
Before processing data extracted from the stream, always check for errors returned by getline() (just as you should for any other input/output (IO) operation utilizingstreams).
If your getline() function (or any IO operation on a stream) has set the associated failbit or badbit, do not process the data. You should not assume the data returned is valid or usable for processing. The eofbit is not required to be checked in the loop since this is a normal return and does not necessarily indicate an error that should prevent processing of the data received.
Learning More
Image via unsplash.com
With so many types of data and files that you encounter in writing applications, there are many different ways to manage data streams and strings. You can gain a great deal of additional information on the best ways to utilize the getline C++ function, including:
- C++ programming books and manuals
- Youtube videos and tutorials
- C++ developer forums and user groups
Investigating each of these resources for insight regarding what the getline C++ function is and how to use it in the development of your application, will prove valuable in developing your C++ skills.
What do you think?