Scanner Java: What is Scanner Class In Java?
On the surface, the question what is a scanner class in Java seems easy. A scanner class is a class in Java that allows the program to see user input. However, for the beginning programmer, this information may not be enough. Therefore, this article will examine the subject in depth.
What is Java: An Introduction to the Language
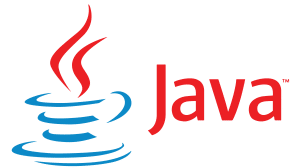
Image via commons.wikimedia.org
While Java has been around for a while, some history may be in order to understand exactly what a scanner class in Java entails. After all, without an understanding of the language, the coder may not realize how crucial the scanner class is to Java programming, and may completely overlook the class's utility.
Java was originally developed by James Gosling in 1991 for use in one of his projects. Interestingly, the language was originally known as “Oak” due to a tree that lived outside of Gosling's office.
After going through iterations such as “woods”, the name “Java” was chosen at random from a list of words. The name “Java” was not planned in the slightest. Tutorialspoint offers an interesting history of Java.
Incidentally, the JavaScript programming language only shares certain features with Java. The two programming languages are not related and confusion between the two can be costly. Code written in Java will not run in a JavaScript environment and vice-versa.
Ultimately, the Java programming language was released in 1995 by Sun Microsystems and became extremely popular for a number of reasons.
First of all, Java is platform independent, meaning that the Java Virtual Machine interprets the code on which ever machine it runs on. Java does not need a separately compiled version of a program for each computer it needs to run on to function properly.
The error checking is also robust, unlike some other popular languages, and Java also supports multi-threading, which almost all modern computers can use to speed processing.
Also, Java is mostly open-source, with much of the source code being released to the general public by Sun in 2006. So, any proficient coder can look at the source code and optimize it or alter it.
Certain other languages, on the other hand, may only be altered by their respective owners, slowing down innovation and creating bottlenecks.
However, possibly the most salient reason for Java's success is that it implements object-oriented programming, which is an increasingly popular category of language among coders and is crucial to understanding the concept of a scanner class in Java.
Why and How Java Uses Classes
As noted above, Java is an object-oriented language. This means that snippets of code are given attributes and treated as objects would be in the physical world.
For example, in the real world, a bicycle may have two wheels, be red and have a horn. In the coding world, these attributes would be represented by classes.
As is always the case with a programming language, objects and classes have a specific naming convention which must be followed.
Generally, in Java, the naming convention is object.class. Please note that other programming languages also follow this convention, most notably JavaScript.
But why do programming languages bother with this? The computer doesn't care about objects or classes, so why would a language bother with being object-based?
Well, the computer may not care, but humans are intrinsic tool-users, so we are used to dealing with objects every day. So, object-oriented programming is an attempt to make programming more natural for humans.
Although object-oriented programming may not seem natural for most people, compared to languages like FORTRAN or COBOL, object-oriented languages are easier to learn.
So now the coder understands a major reason as to Java came to become one of the most popular languages in the world.
The coder should also understand what a class is, and how objects in Java use object-oriented programming in order to make coding concepts easier for coders.
The Scanner Class in Java: A Special Case
However, the scanner class is particular to Java, and the concept should be fully explained. The scanner class is part of the Java util package, which is also important to understand.
The Java util package contains a number of classes that are crucial to effectively working with Java. As always, the naming convention is object.package.class. So when using the util package, the proper nomenclature would be object.util.class.
While this article is concerned with remaining simple, coders who wish to find a more complex definition of scanner class in Java would be advised to visit geeksforgeeks.org
The first, and perhaps most important class is “arrays”. This class allows the Java user to search and sort arrays.
Arrays are often used in programming languages—they essentially are lists of variables that store information, including letters, numbers and even other arrays. Being able to search and sort through arrays is crucial, and this util class allows coders to do just that.
There are many other util classes which are important to all levels of coding.
They include the Dictionary class, which helps map keyboard presses to values, the EventListenerProxy which can aid in getting input from users, and the EventObject class, which helps the coder make something happen onscreen when something else occurs.
However, almost unarguably, the most important class contained in the util package is the scanner class. The scanner class is a simple text parser that allows a program written in Java to “see” primitive types and strings.
In other words, this class allows an object to react to what a user types after the user inputs a string.
This class offers the easiest way for a beginning programmer to add interactivity to their coding.
It is often used, even when there are other alternatives available, due to its utility. However, there are some situations in which another class may be more appropriate.
For example, the scanner class should be avoided when creating an application needing fast input response, such as a game. The class has a slow response time and therefore gaming would not be appropriate.
However, for most other uses, the scanner class excels. General text input is handled very well by this class, and so text input for databases, spreadsheets and other programs is often given to this class in Java.
It's difficult to envision the Java environment being as easy-to-use and successful as it is without the usage of scanner class.
A Sample of Methods for the Scanner Class in Java
Of course, like almost any class, the Scanner class has methods which help define what the scanner is doing at that particular moment. A few of those methods are as follows:
The method listed as close() voids the scanner completely. This method stops whatever the scanner is doing and closes it. This method is obviously useful when the coder wishes for the scanner to completely cease functioning.
Meanwhile, the method listed as FindInLine() is used to find the next sequence constructed from the specified string, ignoring delimiters.
The final example is hasNextBoolean() which looks to see if the text response is a yes or no answer and then respond accordingly. As with all Boolean functions, only yes or no answers are accepted with this method, which is useful in certain situations.
If the reader is interested in a more comprehensive list of methods for the scanner class in Java, Javatpoint has an extensive list, and is highly recommended.
Effectively Using The Scanner Class In Java
As can be seen from the above examples, the scanner class is flexible and useful. However, like most programming tools, there are situations in which the use should be avoided.
For example, if the coder is attempting to create a multithreaded program, choosing to use the Java Scanner class should be avoided unless external synchronization is used.
However, this is no reason to avoid the scanner class in Java. While exceptions do exist, this class is one of the most used in all of Java, and with good reason.
When a coder needs a simple, reliable class for receiving text input from a user, the scanner class should be considered. The prepared coder should be familiar with the scanner class and use it today.
Featured Image via Freepik