How to Fix a Segmentation Fault on Linux
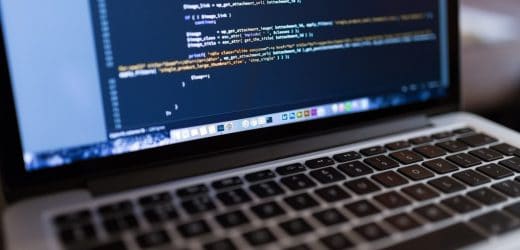
When running applications, whether it’s on your office desktop, home computer, or mobile device, you just expect them to work.
Apps that crash or don’t function properly can be frustrating to users and are certainly
troublesome for developers.
One of the most problematic messages presented to users and programmers on Linux environments is the all-too-familiar “Segmentation Fault.”
This may not be the time to panic, but it can only be resolved easily if you know how to fix a segmentation fault on Linux.
Comparison Table
[amazon box=”B0013JLRH2,B007QO8SA2,1719439559,1484964098,1441917470,1788993861″ template=”table”]
What Is a Segmentation Fault?
A segmentation fault – also abbreviated as segfault – is actually an error related to memory usage. It can mean that your program performed an invalid memory function due to:
- A memory address that does not exist
- A segment of memory that your program does not have authority to
- A program in a library you’re using has accessed memory that it does not have access to
- Attempts to write to memory owned by the operating system
Operating systems such as Linux normally divide system memory into segments. The operating system allocates these segments for use by system functions, as well as making memory available for user-written applications.
When a program attempts to access a segment that it does not have rights to, or reads or writes to a non-existent memory address, the fault occurs.
The challenge is finding the cause of the segmentation fault, and fixing it.
Common causes of segmentation faults include:
- Exceeding the boundary of an array, resulting in a buffer overflow
- Accessing a memory segment that has been deleted
- Referencing memory that has not been allocated for your use
- Attempting to write to read-only memory
- Address pointers that are initialized to null values being dereferenced
Operating systems such as Linux and Unix incorporate memory management techniques that detect such violations of memory use and throw a signal (SIGSEGV, or segmentation violation) to the program that initiated the fault, resulting in your application receiving the dreaded segmentation fault notification.
[amazon link=”B0013JLRH2″ title=”Algorithms and Programming” /]
[amazon box=”B0013JLRH2″]
[amazon link=”B007QO8SA2″ title=”Programming Problems” /]
[amazon box=”B007QO8SA2″]
Causes of Segmentation Faults
The causes and conditions under which segmentation faults take place vary depending on the operating system and even the hardware applications are running on. Underlying
operating system code can detect and recover from some wayward addressing errors created by application errors, isolating system memory from unauthorized destruction or access caused by buffer overflows or inadvertent programming errors.
Part of the problem in dealing with and resolving segmentation faults on Linux is finding the root cause of the problem. Often segfaults happen as a result of application errors that occurred earlier in an application, such as compromising a memory address that will be referenced later in the program.
In such conditions, the segmentation fault can be encountered when the address is utilized, but the cause is in a different area of the program. Backtracking through the
functionality of the program is often the only way to determine the actual cause of the error.
This is especially true when the segmentation fault presents itself intermittently, which may indicate that there is a relationship with a particular segment of programming code that is encountered only under specific conditions.
Often one of the most challenging factors in isolating the cause of a segmentation fault is in reproducing the error in a consistent manner before you can fix the cause of the fault.
Your Best Way to Fix Segmentation Faults On Linux
Your most foolproof method of fixing segmentation faults is to simply avoid them. This may not always be an option, of course.
If you’re running packaged software or applications that you have downloaded from the internet or provided by a friend or business associate, you may be out of luck. One option for packaged software is to submit a problem report to the vendor or supplier, and hope they will provide a solution or fix the problem through an update or replacement.
As a programmer, you should adhere to best practices in memory management:
- Keep close track of memory allocation and deletion
- Diagnose problems thoroughly through adequate testing of all programs and sub-programs
- Utilize tools for debugging that can help you determine the true cause of the segmentation fault
Trouble-shooting memory violations that cause segfault issues can be tricky, without the use of a good debugger, since the code that caused the memory issue may be in a totally different section of your code from where the segmentation fault crashes the program.
Some compilers will detect invalid access to memory locations such as writing to read-only memory, indicating an error that can be corrected before the program is utilized for testing or production use. Unfortunately, there are also compilers that will not highlight such coding errors or will allow the creation of the executable code despite these errors. The addressing error will not be noted until the program is run, and the segmentation fault rears its ugly head.
Debugging can help you locate the exact section or line of code that is causing the error.
[amazon link=”1719439559″ title=”Fundamentals of Programming Terms and Concepts” /]
[amazon box=”1719439559″]
[amazon link=”1484964098″ title=”Programming Problems 2″ /]
[amazon box=”1484964098″]
Finding and Fixing Segmentation Faults On Linux
The typical action that Linux-based systems take in the event of a segmentation fault is to terminate the execution of the offending process that initiated the condition. Along with halting the program or process, a core file or core dump will often be generated, which is an important tool in debugging the program or finding the cause of the segfault.
Core dumps are valuable in locating specific information regarding the process that was running when the segmentation fault occurred:
- Snapshot of program memory at the time of termination
- Program and stack pointers
- Processor register content
- Additional useful memory management and OS information
When system-generated core dumps do not provide adequate information for locating the cause of the problem, you can also force dumps at points in your code, to get an exact picture of addresses and memory content at
any point during execution.
Fixing the Segmentation Fault
Sooner or later, every programmer will encounter a program that produces a segmentation fault, requiring some level of debugging to find the source of the error. There are several ways to go about some simple troubleshooting and debugging of a program:
- Make assumptions about what the program is doing at the point of the segfault, guess what the problem is, and attempt to fix the code to resolve the problem (not very scientific or reliable).
- Change the program to list variables at strategic points, to help pinpoint the issue.
- Utilizing a debugging tool to trap the details of program execution and really nail down the exact cause of the segmentation fault.
What makes the most sense to you? Using a debugger, of course.
GDB is a debugging tool available for Unix-type systems and can be a valuable tool in your programming arsenal. With GDB functions you are able to pinpoint the exact location in your programs where segmentation faults are generated and backtrack to the
root cause with minimal time and effort. GDB functionality includes many important functions:
Start your program under GDB control – now the debugger is running behind the scenes, tracking each step of execution. When the segfault takes place, the debugger supplies you with an abundance of valuable information:
- The line of code where the fault took place
- Details of the program code being executed
Now you have a good clue as to where the problem is, but how did the program get to that point, and what was information was it working with?
Simply tell the debugger to backtrace, and you will have even more information presented:
- The methods that called this statement
- Parameters that were passed
- Variables in use
So now you know how the program got to the point of the segfault, but perhaps not enough to resolve the problem. This is where additional functions of the debugger come into play for additional troubleshooting steps.
You can set breakpoints in your program so that GDB will stop execution at exactly that point of failure in your logic, allowing you to display what was in variables and memory addresses when that breakpoint is reached. Breakpoints can even include conditions, such that you can break only under specific circumstances.
If that’s not quite enough to identify the problem, set the breakpoint a little earlier in your logic, then tell the debugger to “step” through the logic one line at a time, evaluating the variables and memory constants at each step, until you identify exactly where the unexpected values appear.
[amazon link=”1441917470″ title=”Algorithms and Programming” /]
[amazon box=”1441917470″]
[amazon link=”1788993861″ title=”The Modern C++ Challenge” /]
[amazon box=”1788993861″]
Ready to Fix a Segmentation Fault on Linux?
Following the debugging process through your program will nearly always pinpoint the problem that is the root cause of your segmentation faults.
Be certain to follow best practices in your Linux application programming development. Combining good memory management techniques with sophisticated debugging tools will allow you to produce reliable, fault-free programs for use in Linux environments.
.
What do you think?